Spread Beepers Solution
Spread Beepers is a problem in the Stanford Code in Place course. Learn how to use decomposition to solve the problem.
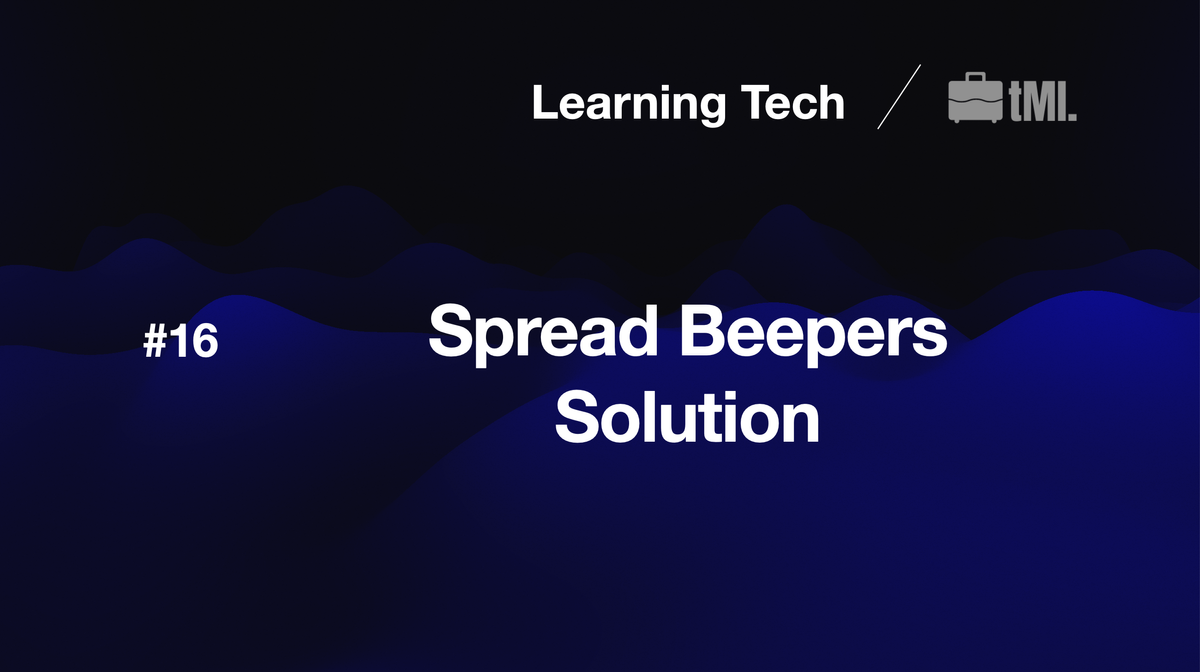
Spread beepers is an interesting problem. While it's easy for a human eye to solve the problem, it actually takes a bit of decomposing skills for Karel to finish the job.
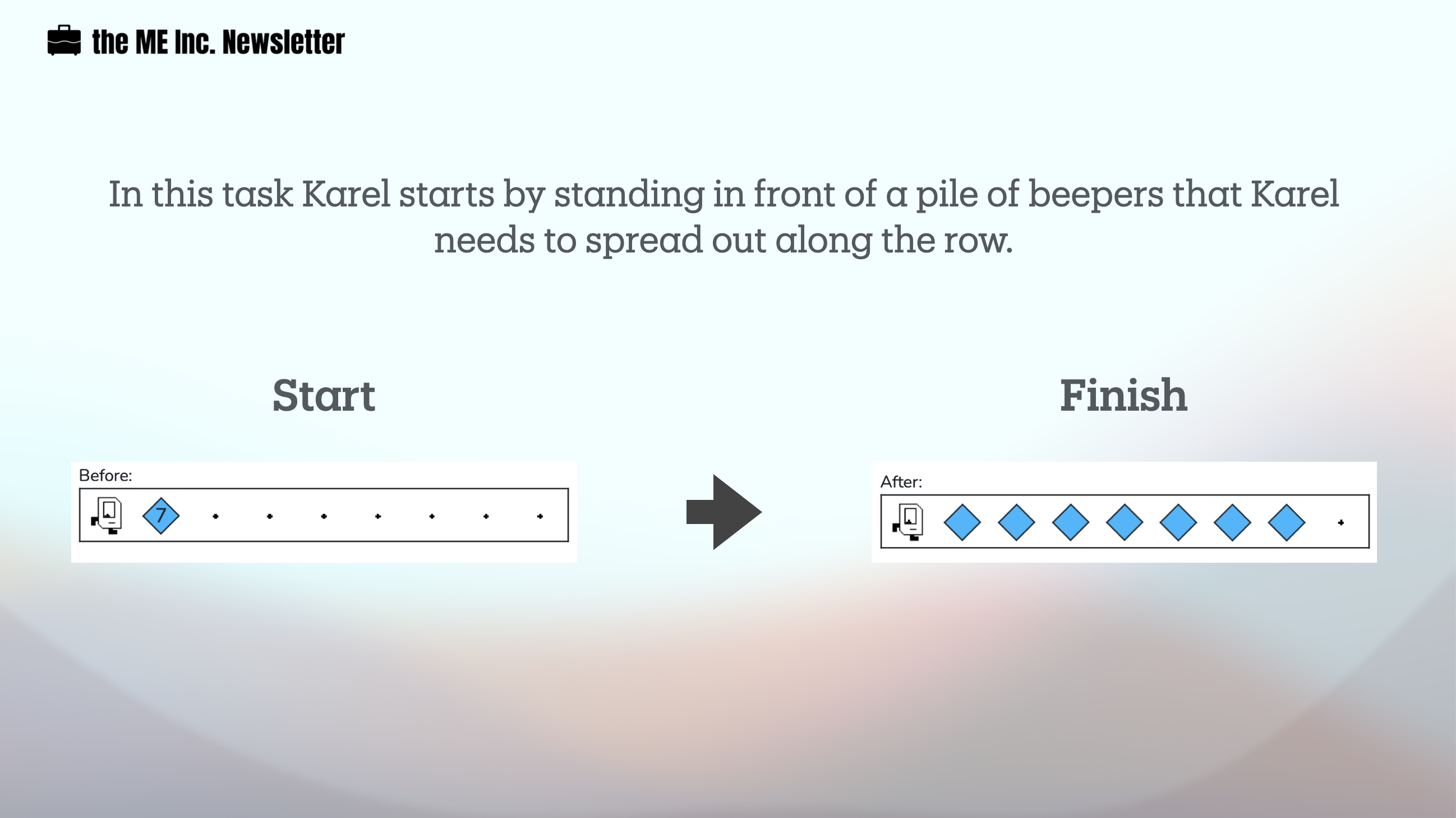
Karel has the ability to find out whether there are beepers present, but she cannot know how many left. In other words, Karel doesn't count.
The Thinking
Since the pile of beepers is always on the second corner, Karel should move just once to the beepers location. After that, Karel can start doing her work. Since Karel already possesses infinite numbers of beepers in her bag, and Karel doesn't know how to count, she cannot pick up all the beepers at once. Instead, Karel should pick up a beeper, go to the next empty location, put down the beeper, and return to the starting corner, facing east.
One of the most difficult issue with this problem is that since Karel doesn't know how to count, she will keep picking up beeper even when there is only one left. Our solution to this issue is to put the beeper back if Karel incorrectly picks up the last beeper from the second corner. To accomplish this, we will have to put in an additional layer of condition.
def spread():
while beepers_present():
pick_beeper() # karel can only pick
if beepers_present():
move_to_next()
put_beeper()
move_to_start()
move()
put_beeper()
def turn_back():
turn_left()
turn_left()
def move_to_start():
turn_back()
while front_is_clear():
move()
turn_back()
def move_to_next():
while beepers_present():
move()
The while loop and the if loop together helps Karel understand when she needs to stop picking up beepers. If you understand this, the solution become natural.
The Coding
from karel.stanfordkarel import *
# let it go to starting point at the end
## helper function
## 1 turn back
def turn_back():
turn_left()
turn_left()
## 2 move to the start
def move_to_start():
turn_back()
while front_is_clear():
move()
turn_back()
## 3 move to next
def move_to_next():
while beepers_present():
move()
## 4 work function find and spread beeper
def spread():
while beepers_present():
pick_beeper() # karel can only pick
if beepers_present():
move_to_next()
put_beeper()
move_to_start()
move()
put_beeper()
## Main
def main():
move()
spread()
move_to_start()
# There is no need to edit code beyond this point
if __name__ == '__main__':
main()